An Introduction to TypeScript
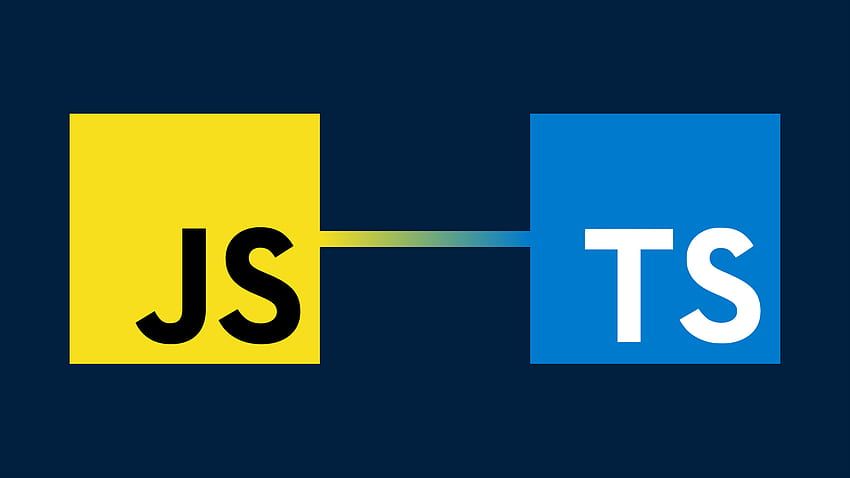
TypeScript is a language that was developed by Microsoft as a superset of JavaScript. It was designed to address some of the shortcomings of JavaScript and make it easier to write large-scale, complex applications. It's become increasingly popular among developers in recent years, and for good reason.
Benefits of TypeScript
One of the primary benefits of TypeScript is its strong typing system. In JavaScript, you don't have to declare the type of a variable before using it, which can make debugging more difficult. With TypeScript, you can declare the type of a variable, which can help catch errors before your code is executed. This can save you time and frustration in the long run.
Another benefit of TypeScript is its support for classes and interfaces. These features allow you to define complex data structures and enforce type checking on them. This can make your code more maintainable and easier to read.
TypeScript also includes many of the features of ECMAScript 6 (ES6), the latest version of JavaScript. This means that you can use features like arrow functions, destructuring, and spread operators in your TypeScript code. Additionally, TypeScript includes its own features, such as decorators, which allow you to modify the behavior of classes and functions.
One of the most powerful features of TypeScript is its ability to catch errors before you run your code. The TypeScript compiler will analyze your code and alert you to potential issues before you ever execute your program. This can save you time and headaches, as you can fix issues before they become major problems.
TypeScript is also compatible with many popular JavaScript libraries and frameworks. This means that you can use TypeScript with libraries like React and Angular, which can make your code more robust and easier to maintain.
In addition to its benefits for developers, TypeScript is also popular among companies. Its strong typing system and other features make it easier to write maintainable, scalable code. This can save companies time and money in the long run, as they can avoid costly errors and quickly make changes to their codebase
Why use TypeScript?
TypeScript has many benefits for web development. Some of them are:
- TypeScript helps to write better code, with fewer errors and bugs. By using types and other features, developers can avoid common mistakes such as typos, null references, undefined values, etc.
- TypeScript makes code more readable and maintainable. By using types and other features, developers can document their code better and make it easier to understand for themselves and others.
- TypeScript enables code reuse and modularity. By using modules and interfaces, developers can organize their code into reusable components and avoid duplication.
- TypeScript facilitates code refactoring and testing. By using types and other features,
developers can refactor their code safely and efficiently without breaking functionality. They can also use tools such as Jest or Mocha to test their code with confidence. - TypeScript supports modern web development frameworks such as Angular,
React,
Vue,
and others. These frameworks use TypeScript as their preferred language or provide support for it.
How to get started with TypeScript?
To get started with TypeScript, you need to install Node.js and npm on your machine.
Then, you can install TypeScript globally using this command:
npm install -g typescript
You can also install TypeScript locally in your project folder using this command:
npm install --save-dev typescript
To create a simple TypeScript file, you can use any text editor or IDE that supports TypeScript, such as Visual Studio Code or WebStorm (to name but a few).
You can name your file with a .ts
extension, such as hello.ts
.
Here is an example of a simple TypeScript file:
// hello.ts
function greet(name: string) {
console.log(`Hello ${name}!`);
}
greet("World");
To compile this file to JavaScript, you can use this command:
tsc hello.ts
This will create a file called hello.js
in the same folder, which contains the compiled JavaScript code:
// hello.js
function greet(name) {
console.log("Hello " + name + "!");
}
greet("World");
You can run this file using Node.js or any browser that supports JavaScript.
TLDR;
TypeScript is a powerful programming language that enhances JavaScript with types and other features.
It helps developers write better, more readable, and more scalable web applications.
If you want to learn more about TypeScript, you can visit its official website, where you will find documentation, tutorials, examples, and more resources.